java制作坦克大战TankWar
如果您发现本文排版有问题,可以先点击下面的链接切换至老版进行查看!!!
java制作坦克大战TankWar
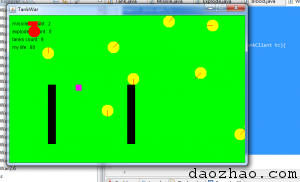
- 分类:
- Java
更新时间:
上一篇:j2se java编写简易聊天室程序下一篇:今天完成java坦克大战
相关文章
分享个儿时的90坦克javascript版
效果图如下 首先是主页面tanke.php [code lang="php"] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 阅读更多…
今天完成java坦克大战
今天练习了几个java版的坦克大战,有单机版,图片版,网络版。继续练习其它java程序。 阅读更多…